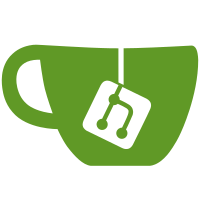
Some checks failed
NPM Installation / build (16.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (16.x, windows-latest) (push) Has been cancelled
NPM Installation / build (17.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (17.x, windows-latest) (push) Has been cancelled
NPM Installation / build (18.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (18.x, windows-latest) (push) Has been cancelled
146 lines
4.0 KiB
JavaScript
146 lines
4.0 KiB
JavaScript
import React, { useEffect, useState } from 'react'
|
|
import {
|
|
Table,
|
|
TableBody,
|
|
TableCell,
|
|
TableContainer,
|
|
TableHead,
|
|
TableRow,
|
|
Paper,
|
|
TextField,
|
|
Button,
|
|
Typography,
|
|
TablePagination,
|
|
Box,
|
|
} from '@mui/material'
|
|
import axios from 'axios'
|
|
import { isAutheticated } from '../../../auth'
|
|
import Axios from '../../../axios'
|
|
import Swal from 'sweetalert2'
|
|
|
|
function StockTable() {
|
|
const [stocks, setStocks] = useState([]) // Store stock data
|
|
const [loading, setLoading] = useState(true) // Loading state
|
|
const [rowsPerPage, setRowsPerPage] = useState(5)
|
|
const [page, setPage] = useState(0)
|
|
const token = isAutheticated()
|
|
|
|
// Fetch stock data from the backend
|
|
const fetchStocks = async () => {
|
|
try {
|
|
const response = await Axios.get('/api/pd/stock', {
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
})
|
|
setStocks(response.data.stocks || [])
|
|
} catch (error) {
|
|
console.error('Error fetching stocks:', error)
|
|
} finally {
|
|
setLoading(false)
|
|
}
|
|
}
|
|
|
|
// Handle stock value change and persist across pagination
|
|
const handleStockChange = (productId, value) => {
|
|
setStocks((prevStocks) =>
|
|
prevStocks.map((stock) =>
|
|
stock.productid === productId ? { ...stock, Stock: parseInt(value, 10) || 0 } : stock,
|
|
),
|
|
)
|
|
}
|
|
console.log(stocks, 'stocks')
|
|
|
|
// Submit updated stock values
|
|
const handleSubmit = async () => {
|
|
try {
|
|
await Axios.put(
|
|
'/api/pd/stock-update',
|
|
{ products: stocks },
|
|
{
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
},
|
|
)
|
|
Swal.fire('success!', 'Stock updated successfully', 'success')
|
|
} catch (error) {
|
|
console.error('Error updating stock:', error)
|
|
Swal.fire('error!', 'Something went wrong', 'error')
|
|
}
|
|
}
|
|
|
|
// Pagination handlers
|
|
const handleChangePage = (event, newPage) => setPage(newPage)
|
|
const handleChangeRowsPerPage = (event) => {
|
|
setRowsPerPage(parseInt(event.target.value, 10))
|
|
setPage(0)
|
|
}
|
|
|
|
// Fetch stocks on component mount
|
|
useEffect(() => {
|
|
fetchStocks()
|
|
}, [])
|
|
|
|
return (
|
|
<Box p={3}>
|
|
<Typography variant="h5" mb={2}>
|
|
Product Stock Management
|
|
</Typography>
|
|
<TableContainer component={Paper}>
|
|
<Table>
|
|
<TableHead>
|
|
<TableRow>
|
|
<TableCell>Product Name</TableCell>
|
|
<TableCell>SKU</TableCell>
|
|
<TableCell>Current Stock</TableCell>
|
|
</TableRow>
|
|
</TableHead>
|
|
<TableBody>
|
|
{loading ? (
|
|
<TableRow>
|
|
<TableCell colSpan={3} align="center">
|
|
<Typography>Loading...</Typography>
|
|
</TableCell>
|
|
</TableRow>
|
|
) : (
|
|
stocks.slice(page * rowsPerPage, page * rowsPerPage + rowsPerPage).map((stock) => (
|
|
<TableRow key={stock.productid}>
|
|
<TableCell>{stock.name}</TableCell>
|
|
<TableCell>{stock.SKU}</TableCell>
|
|
<TableCell>
|
|
<TextField
|
|
type="number"
|
|
value={stock.Stock}
|
|
onChange={(e) => handleStockChange(stock.productid, e.target.value)}
|
|
variant="outlined"
|
|
size="small"
|
|
/>
|
|
</TableCell>
|
|
</TableRow>
|
|
))
|
|
)}
|
|
</TableBody>
|
|
</Table>
|
|
<TablePagination
|
|
component="div"
|
|
count={stocks.length}
|
|
page={page}
|
|
onPageChange={handleChangePage}
|
|
rowsPerPage={rowsPerPage}
|
|
onRowsPerPageChange={handleChangeRowsPerPage}
|
|
/>
|
|
</TableContainer>
|
|
<Box mt={2} display="flex" justifyContent="flex-end">
|
|
<Button variant="contained" color="primary" onClick={handleSubmit}>
|
|
Update Stock
|
|
</Button>
|
|
</Box>
|
|
</Box>
|
|
)
|
|
}
|
|
|
|
export default StockTable
|