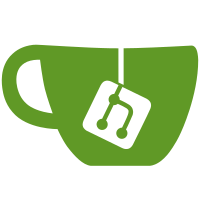
Some checks failed
NPM Installation / build (16.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (16.x, windows-latest) (push) Has been cancelled
NPM Installation / build (17.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (17.x, windows-latest) (push) Has been cancelled
NPM Installation / build (18.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (18.x, windows-latest) (push) Has been cancelled
87 lines
3.0 KiB
JavaScript
87 lines
3.0 KiB
JavaScript
import { Box, Typography, Card, CardContent, Grid } from '@mui/material'
|
|
import React, { useState, useEffect } from 'react'
|
|
import Axios from '../../axios'
|
|
import AddShoppingCartIcon from '@mui/icons-material/AddShoppingCart'
|
|
import LocalShippingIcon from '@mui/icons-material/LocalShipping'
|
|
import CancelIcon from '@mui/icons-material/Cancel'
|
|
import HourglassEmptyIcon from '@mui/icons-material/HourglassEmpty'
|
|
import CheckCircleIcon from '@mui/icons-material/CheckCircle'
|
|
import { isAutheticated } from '../../auth'
|
|
const Dashboard = () => {
|
|
const [counts, setCounts] = useState({
|
|
new: 0,
|
|
dispatched: 0,
|
|
cancelled: 0,
|
|
processing: 0,
|
|
delivered: 0,
|
|
})
|
|
const token = isAutheticated()
|
|
// Fetch counts on component mount
|
|
useEffect(() => {
|
|
const getCounts = async () => {
|
|
try {
|
|
const res = await Axios.get('/api/get-counts-pdOrders', {
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
},
|
|
}) // Updated API endpoint
|
|
if (res.status === 200) {
|
|
setCounts(res.data.counts) // Assuming the response is in the format { new, dispatched, cancelled, processing, delivered }
|
|
}
|
|
} catch (error) {
|
|
console.error('Failed to fetch status counts:', error)
|
|
}
|
|
}
|
|
getCounts()
|
|
}, [])
|
|
|
|
// Define colors for different statuses
|
|
const statusColors = {
|
|
new: '#4caf50', // Green
|
|
dispatched: '#2196f3', // Blue
|
|
cancelled: '#f44336', // Red
|
|
processing: '#ff9800', // Orange
|
|
delivered: '#9c27b0', // Purple
|
|
pending: '#56F000 ',
|
|
}
|
|
const statusIcons = {
|
|
new: <AddShoppingCartIcon sx={{ fontSize: 50, color: '#fff' }} />,
|
|
dispatched: <LocalShippingIcon sx={{ fontSize: 50, color: '#fff' }} />,
|
|
cancelled: <CancelIcon sx={{ fontSize: 50, color: '#fff' }} />,
|
|
processing: <HourglassEmptyIcon sx={{ fontSize: 50, color: '#fff' }} />,
|
|
delivered: <CheckCircleIcon sx={{ fontSize: 50, color: '#fff' }} />,
|
|
}
|
|
|
|
return (
|
|
<Box sx={{ flexGrow: 1, padding: 2 }}>
|
|
<Typography textAlign="center" variant="h3" gutterBottom>
|
|
Orders Status Summary
|
|
</Typography>
|
|
|
|
<Grid container spacing={3}>
|
|
{Object.keys(counts).map((status) => (
|
|
<Grid item xs={12} sm={6} md={3} key={status}>
|
|
<Card sx={{ backgroundColor: statusColors[status], color: '#fff' }}>
|
|
<CardContent
|
|
sx={{ display: 'flex', alignItems: 'center', justifyContent: 'space-between' }}
|
|
>
|
|
<Box>
|
|
<Typography variant="h6" component="div">
|
|
{status.charAt(0).toUpperCase() + status.slice(1)}
|
|
</Typography>
|
|
<Typography variant="h4" component="div">
|
|
{counts[status]}
|
|
</Typography>
|
|
</Box>
|
|
{statusIcons[status]} {/* Display the corresponding icon */}
|
|
</CardContent>
|
|
</Card>
|
|
</Grid>
|
|
))}
|
|
</Grid>
|
|
</Box>
|
|
)
|
|
}
|
|
|
|
export default Dashboard
|