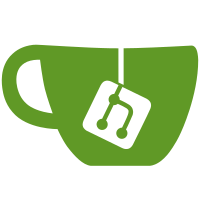
Some checks failed
NPM Installation / build (16.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (16.x, windows-latest) (push) Has been cancelled
NPM Installation / build (17.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (17.x, windows-latest) (push) Has been cancelled
NPM Installation / build (18.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (18.x, windows-latest) (push) Has been cancelled
159 lines
5.1 KiB
JavaScript
159 lines
5.1 KiB
JavaScript
import React, { useEffect, useState } from 'react'
|
|
import {
|
|
Table,
|
|
TableBody,
|
|
TableCell,
|
|
TableContainer,
|
|
TableHead,
|
|
TableRow,
|
|
Paper,
|
|
Typography,
|
|
Skeleton,
|
|
Box,
|
|
Button,
|
|
TablePagination,
|
|
} from '@mui/material'
|
|
import Axios from '../../axios'
|
|
import { isAutheticated } from '../../auth'
|
|
import { useNavigate } from 'react-router-dom'
|
|
|
|
const Order = () => {
|
|
const [orders, setOrders] = useState([])
|
|
const [loading, setLoading] = useState(true)
|
|
const [page, setPage] = useState(0)
|
|
const [rowsPerPage, setRowsPerPage] = useState(5)
|
|
const [totalOrders, setTotalOrders] = useState(0)
|
|
const token = isAutheticated()
|
|
const navigate = useNavigate()
|
|
|
|
const formatAMPM = (date) => {
|
|
var hours = new Date(date).getHours()
|
|
var minutes = new Date(date).getMinutes()
|
|
var ampm = hours >= 12 ? 'PM' : 'AM'
|
|
hours = hours % 12
|
|
hours = hours ? hours : 12 // the hour '0' should be '12'
|
|
minutes = minutes < 10 ? '0' + minutes : minutes
|
|
var strTime = hours + ':' + minutes + ' ' + ampm
|
|
return strTime
|
|
}
|
|
|
|
// Helper function to capitalize the first letter of a string
|
|
const capitalizeFirstLetter = (string) => {
|
|
return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase()
|
|
}
|
|
|
|
useEffect(() => {
|
|
// Fetch orders from the API with pagination
|
|
const fetchOrders = async () => {
|
|
try {
|
|
const response = await Axios.get('/api/get-placed-order-pd', {
|
|
headers: {
|
|
'Access-Control-Allow-Origin': '*',
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
params: {
|
|
page: page + 1, // page number for API (usually starts from 1)
|
|
limit: rowsPerPage, // number of rows per page
|
|
},
|
|
})
|
|
setOrders(response.data.plcaedOrders)
|
|
setTotalOrders(response.data.totalOrders) // Ensure the API returns the total count of orders
|
|
} catch (error) {
|
|
console.error('Error fetching orders:', error)
|
|
} finally {
|
|
setLoading(false)
|
|
}
|
|
}
|
|
|
|
fetchOrders()
|
|
}, [page, rowsPerPage])
|
|
|
|
const handleChangePage = (event, newPage) => {
|
|
setPage(newPage)
|
|
}
|
|
|
|
const handleChangeRowsPerPage = (event) => {
|
|
setRowsPerPage(parseInt(event.target.value, 10))
|
|
setPage(0) // Reset page to 0 when rows per page changes
|
|
}
|
|
|
|
return (
|
|
<Box>
|
|
<Typography variant="h4" mb={2} textAlign={'center'}>
|
|
Order placed list
|
|
</Typography>
|
|
<TableContainer component={Paper}>
|
|
<Table>
|
|
<TableHead>
|
|
<TableRow sx={{ fontWeight: 'bold' }}>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Order ID</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Order Date</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Items</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Order Value</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Status</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Action</TableCell>
|
|
</TableRow>
|
|
</TableHead>
|
|
<TableBody>
|
|
{loading ? (
|
|
Array.from(new Array(rowsPerPage)).map((_, index) => (
|
|
<TableRow key={index}>
|
|
<TableCell colSpan={6}>
|
|
<Skeleton height={40} />
|
|
</TableCell>
|
|
</TableRow>
|
|
))
|
|
) : orders.length > 0 ? (
|
|
orders.map((order) => (
|
|
<TableRow key={order._id}>
|
|
<TableCell>{order.uniqueId}</TableCell>
|
|
<TableCell>
|
|
{new Date(order?.createdAt)
|
|
.toLocaleDateString('en-US', {
|
|
year: 'numeric',
|
|
month: 'short',
|
|
day: 'numeric',
|
|
})
|
|
.replace(',', '')}{' '}
|
|
, {`${formatAMPM(order?.createdAt)}`}
|
|
</TableCell>
|
|
<TableCell>{order.orderItem.length}</TableCell>
|
|
<TableCell>{order.grandTotal.toFixed(2)}</TableCell>
|
|
<TableCell>{capitalizeFirstLetter(order.status)}</TableCell>
|
|
<TableCell>
|
|
<Button
|
|
variant="contained"
|
|
color="primary"
|
|
onClick={() => navigate(`/orders-placed/${order._id}`)}
|
|
>
|
|
View
|
|
</Button>
|
|
</TableCell>
|
|
</TableRow>
|
|
))
|
|
) : (
|
|
<TableRow>
|
|
<TableCell colSpan={6} align="center">
|
|
<Typography variant="body1">Data not found</Typography>
|
|
</TableCell>
|
|
</TableRow>
|
|
)}
|
|
</TableBody>
|
|
</Table>
|
|
<TablePagination
|
|
rowsPerPageOptions={[5, 10, 25]}
|
|
component="div"
|
|
count={totalOrders} // Total number of orders
|
|
rowsPerPage={rowsPerPage}
|
|
page={page}
|
|
onPageChange={handleChangePage}
|
|
onRowsPerPageChange={handleChangeRowsPerPage}
|
|
/>
|
|
</TableContainer>
|
|
</Box>
|
|
)
|
|
}
|
|
|
|
export default Order
|