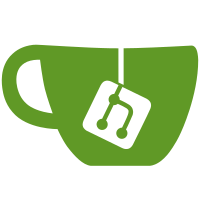
Some checks failed
NPM Installation / build (16.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (16.x, windows-latest) (push) Has been cancelled
NPM Installation / build (17.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (17.x, windows-latest) (push) Has been cancelled
NPM Installation / build (18.x, ubuntu-latest) (push) Has been cancelled
NPM Installation / build (18.x, windows-latest) (push) Has been cancelled
352 lines
11 KiB
JavaScript
352 lines
11 KiB
JavaScript
import React, { useState, useEffect } from 'react'
|
|
import {
|
|
Box,
|
|
Table,
|
|
TableBody,
|
|
TableCell,
|
|
TableContainer,
|
|
TableHead,
|
|
TableRow,
|
|
Paper,
|
|
TablePagination,
|
|
Button,
|
|
Tooltip,
|
|
Tabs,
|
|
Tab,
|
|
Modal,
|
|
TextField,
|
|
DialogActions,
|
|
Typography,
|
|
} from '@mui/material'
|
|
import { useNavigate } from 'react-router-dom'
|
|
import Axios from '../../../axios'
|
|
import { isAutheticated } from '../../../auth'
|
|
import Swal from 'sweetalert2'
|
|
|
|
const Kyc = () => {
|
|
const [rows, setRows] = useState([])
|
|
const [page, setPage] = useState(0)
|
|
const [rowsPerPage, setRowsPerPage] = useState(10)
|
|
const [tabIndex, setTabIndex] = useState(0)
|
|
const [loading, setLoading] = useState(false)
|
|
const [openModal, setOpenModal] = useState(false)
|
|
const [openModal2, setOpenModal2] = useState(false)
|
|
const [rejectionReason, setRejectionReason] = useState('')
|
|
const [selectedRowId, setSelectedRowId] = useState(null)
|
|
const [selectedRowId2, setSelectedRowId2] = useState(null)
|
|
const navigate = useNavigate()
|
|
const token = isAutheticated()
|
|
|
|
useEffect(() => {
|
|
const fetchData = async () => {
|
|
try {
|
|
const response = await Axios.get('/api/kyc/getAll/', {
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
})
|
|
console.log(response)
|
|
setRows(response.data)
|
|
} catch (error) {
|
|
console.error('Error fetching data: ', error)
|
|
}
|
|
}
|
|
|
|
fetchData()
|
|
}, [])
|
|
|
|
const handleChangePage = (event, newPage) => {
|
|
setPage(newPage)
|
|
}
|
|
|
|
const handleChangeRowsPerPage = (event) => {
|
|
setRowsPerPage(parseInt(event.target.value, 10))
|
|
setPage(0)
|
|
}
|
|
|
|
const handleViewClick = (id) => {
|
|
navigate(`/kyc/details/${id}`)
|
|
}
|
|
|
|
const handleTabChange = (event, newValue) => {
|
|
setTabIndex(newValue)
|
|
}
|
|
|
|
const filterRowsByStatus = (status) => {
|
|
return rows.filter((row) => row.status === status)
|
|
}
|
|
|
|
const filteredRows = filterRowsByStatus(
|
|
tabIndex === 0 ? 'new' : tabIndex === 1 ? 'approved' : 'reject',
|
|
)
|
|
|
|
const handleRejectClick = async (id) => {
|
|
setSelectedRowId(id)
|
|
setOpenModal(true)
|
|
}
|
|
|
|
const handleApproveClick = async (id) => {
|
|
setSelectedRowId2(id)
|
|
setOpenModal2(true)
|
|
}
|
|
|
|
const handleApproveConfirm = async () => {
|
|
try {
|
|
setLoading(true)
|
|
console.log(selectedRowId2)
|
|
await Axios.patch(
|
|
`/api/kyc/update/${selectedRowId2}`,
|
|
{
|
|
status: 'approved',
|
|
},
|
|
{
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
},
|
|
)
|
|
setRows((prevRows) =>
|
|
prevRows.map((row) => (row._id === selectedRowId2 ? { ...row, status: 'approved' } : row)),
|
|
)
|
|
setLoading(true)
|
|
Swal.fire('Success', 'Approved', 'success')
|
|
handleModalClose2()
|
|
} catch (error) {
|
|
setLoading(false)
|
|
console.error('Error approving KYC: ', error)
|
|
Swal.fire('Error!', error.message, 'error')
|
|
handleModalClose2()
|
|
}
|
|
}
|
|
|
|
const handleModalClose = () => {
|
|
setOpenModal(false)
|
|
setRejectionReason('')
|
|
}
|
|
|
|
const handleModalClose2 = () => {
|
|
setOpenModal2(false)
|
|
}
|
|
|
|
const handleRejectionReasonChange = (event) => {
|
|
setRejectionReason(event.target.value)
|
|
}
|
|
|
|
const handleRejectConfirm = async () => {
|
|
try {
|
|
setLoading(true)
|
|
await Axios.patch(
|
|
`/api/kyc/update/${selectedRowId}`,
|
|
{
|
|
status: 'reject',
|
|
rejectionReason,
|
|
user: 'Principal Distributer',
|
|
},
|
|
{
|
|
headers: {
|
|
Authorization: `Bearer ${token}`,
|
|
'Content-Type': 'application/json',
|
|
},
|
|
},
|
|
)
|
|
setRows((prevRows) =>
|
|
prevRows.map((row) => (row._id === selectedRowId ? { ...row, status: 'reject' } : row)),
|
|
)
|
|
setLoading(true)
|
|
Swal.fire('Success', 'Rejected', 'success')
|
|
handleModalClose()
|
|
} catch (error) {
|
|
setLoading(false)
|
|
console.error('Error rejecting KYC: ', error)
|
|
Swal.fire('Error!', error.message, 'error')
|
|
handleModalClose()
|
|
}
|
|
}
|
|
|
|
const formatAMPM = (date) => {
|
|
var hours = new Date(date).getHours()
|
|
var minutes = new Date(date).getMinutes()
|
|
var ampm = hours >= 12 ? 'PM' : 'AM'
|
|
hours = hours % 12
|
|
hours = hours ? hours : 12 // the hour '0' should be '12'
|
|
minutes = minutes < 10 ? '0' + minutes : minutes
|
|
var strTime = hours + ':' + minutes + ' ' + ampm
|
|
return strTime
|
|
}
|
|
const capitalizeStatus = (status) => {
|
|
return status.charAt(0).toUpperCase() + status.slice(1)
|
|
}
|
|
|
|
// Helper function to format the date
|
|
const formatDateWithoutComma = (date) => {
|
|
const dateObj = new Date(date)
|
|
const dateStr = dateObj.toDateString().split(' ')
|
|
return `${dateStr[1]} ${dateStr[2]} ${dateStr[3]}`
|
|
}
|
|
return (
|
|
<Box sx={{ width: '100%' }}>
|
|
<Paper sx={{ width: '100%', mb: 2 }}>
|
|
<Tabs value={tabIndex} onChange={handleTabChange}>
|
|
<Tab label="New" sx={{ textTransform: 'unset' }} />
|
|
<Tab label="Approved" sx={{ textTransform: 'unset' }} />
|
|
<Tab label="Rejected" sx={{ textTransform: 'unset' }} />
|
|
</Tabs>
|
|
<TableContainer>
|
|
<Table>
|
|
<TableHead>
|
|
<TableRow>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>ID</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Trade Name</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Created On</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Status</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Action</TableCell>
|
|
</TableRow>
|
|
</TableHead>
|
|
<TableBody>
|
|
{filteredRows.length !== 0 &&
|
|
filteredRows
|
|
.slice(page * rowsPerPage, page * rowsPerPage + rowsPerPage)
|
|
.map((row) => (
|
|
<TableRow key={row._id}>
|
|
<TableCell>{row._id}</TableCell>
|
|
<TableCell>{row.trade_name}</TableCell>
|
|
<TableCell>
|
|
{`${formatDateWithoutComma(row?.createdAt)}`}
|
|
<span>, {`${formatAMPM(row?.createdAt)}`}</span>
|
|
</TableCell>
|
|
<TableCell>{capitalizeStatus(row.status)}</TableCell>
|
|
<TableCell>
|
|
<Tooltip title="View">
|
|
<Button
|
|
sx={{ mr: '1rem', textTransform: 'unset' }}
|
|
color="primary"
|
|
variant="contained"
|
|
onClick={() => handleViewClick(row._id)}
|
|
>
|
|
View
|
|
</Button>
|
|
</Tooltip>
|
|
{tabIndex === 0 && (
|
|
<>
|
|
<Tooltip title="Approve">
|
|
<Button
|
|
sx={{ mr: '1rem', textTransform: 'unset' }}
|
|
color="success"
|
|
variant="contained"
|
|
onClick={() => handleApproveClick(row._id)}
|
|
>
|
|
Approve
|
|
</Button>
|
|
</Tooltip>
|
|
<Tooltip title="Reject">
|
|
<Button
|
|
sx={{ mr: '1rem', textTransform: 'unset' }}
|
|
color="error"
|
|
variant="contained"
|
|
onClick={() => handleRejectClick(row._id)}
|
|
>
|
|
Reject
|
|
</Button>
|
|
</Tooltip>
|
|
</>
|
|
)}
|
|
</TableCell>
|
|
</TableRow>
|
|
))}
|
|
{filteredRows.length === 0 && (
|
|
<h5 style={{ textAlign: 'center' }}>No kyc available</h5>
|
|
)}
|
|
</TableBody>
|
|
</Table>
|
|
</TableContainer>
|
|
<TablePagination
|
|
rowsPerPageOptions={[10, 15, 25]}
|
|
component="div"
|
|
count={filteredRows.length}
|
|
rowsPerPage={rowsPerPage}
|
|
page={page}
|
|
onPageChange={handleChangePage}
|
|
onRowsPerPageChange={handleChangeRowsPerPage}
|
|
/>
|
|
</Paper>
|
|
<Modal
|
|
open={openModal}
|
|
onClose={handleModalClose}
|
|
aria-labelledby="modal-modal-title"
|
|
aria-describedby="modal-modal-description"
|
|
>
|
|
<Box sx={{ ...modalStyle, width: 400 }}>
|
|
<h2 id="modal-modal-title">Rejection Reason</h2>
|
|
<TextField
|
|
fullWidth
|
|
multiline
|
|
rows={4}
|
|
value={rejectionReason}
|
|
onChange={handleRejectionReasonChange}
|
|
variant="outlined"
|
|
label="Enter rejection reason"
|
|
/>
|
|
<DialogActions>
|
|
<Button onClick={handleModalClose} sx={{ textTransform: 'unset' }} color="primary">
|
|
Cancel
|
|
</Button>
|
|
<Button
|
|
onClick={handleRejectConfirm}
|
|
sx={{ textTransform: 'unset' }}
|
|
color="error"
|
|
variant="contained"
|
|
>
|
|
Confirm
|
|
</Button>
|
|
</DialogActions>
|
|
</Box>
|
|
</Modal>
|
|
<Modal
|
|
open={openModal2}
|
|
onClose={handleModalClose2}
|
|
aria-labelledby="modal-modal-title"
|
|
aria-describedby="modal-modal-description"
|
|
>
|
|
<Box sx={{ ...modalStyle, width: 400 }}>
|
|
<h2 id="modal-modal-title">Approval Confirmation</h2>
|
|
<Typography id="modal-modal-description" sx={{ mt: 2 }}>
|
|
Are you sure you want to approve this KYC?
|
|
</Typography>
|
|
<DialogActions>
|
|
<Button
|
|
onClick={handleModalClose2}
|
|
sx={{ textTransform: 'unset' }}
|
|
color="primary"
|
|
// variant="contained"
|
|
>
|
|
Cancel
|
|
</Button>
|
|
<Button
|
|
onClick={handleApproveConfirm}
|
|
sx={{ textTransform: 'unset' }}
|
|
color="success"
|
|
variant="contained"
|
|
>
|
|
Confirm
|
|
</Button>
|
|
</DialogActions>
|
|
</Box>
|
|
</Modal>
|
|
</Box>
|
|
)
|
|
}
|
|
|
|
const modalStyle = {
|
|
position: 'absolute',
|
|
top: '50%',
|
|
left: '50%',
|
|
transform: 'translate(-50%, -50%)',
|
|
bgcolor: 'background.paper',
|
|
boxShadow: 24,
|
|
p: 4,
|
|
}
|
|
|
|
export default Kyc
|