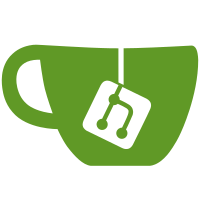
Some checks are pending
NPM Installation / build (16.x, ubuntu-latest) (push) Waiting to run
NPM Installation / build (16.x, windows-latest) (push) Waiting to run
NPM Installation / build (17.x, ubuntu-latest) (push) Waiting to run
NPM Installation / build (17.x, windows-latest) (push) Waiting to run
NPM Installation / build (18.x, ubuntu-latest) (push) Waiting to run
NPM Installation / build (18.x, windows-latest) (push) Waiting to run
118 lines
3.1 KiB
JavaScript
118 lines
3.1 KiB
JavaScript
import React, { useEffect, useState, useRef } from 'react'
|
|
import Axios from '../../../axios'
|
|
import { isAutheticated } from '../../../auth'
|
|
import {
|
|
Box,
|
|
Table,
|
|
TableBody,
|
|
TableCell,
|
|
TableContainer,
|
|
TableHead,
|
|
TableRow,
|
|
Paper,
|
|
Button,
|
|
TextField,
|
|
CircularProgress,
|
|
Pagination,
|
|
} from '@mui/material'
|
|
import { Link } from 'react-router-dom'
|
|
|
|
const ProductManual = () => {
|
|
const [loading, setLoading] = useState(true)
|
|
const token = isAutheticated()
|
|
const [productManuals, setProductManuals] = useState([])
|
|
const [currentPage, setCurrentPage] = useState(1)
|
|
const [itemsPerPage, setItemsPerPage] = useState(10)
|
|
const [totalData, setTotalData] = useState(0)
|
|
const titleRef = useRef('')
|
|
|
|
const getProductManuals = async () => {
|
|
try {
|
|
setLoading(true)
|
|
const response = await Axios.get(`/api/productmanual/getall`, {
|
|
headers: { Authorization: `Bearer ${token}` },
|
|
params: {
|
|
page: currentPage,
|
|
show: itemsPerPage,
|
|
title: titleRef.current.value || '',
|
|
},
|
|
})
|
|
if (response.status === 200) {
|
|
const { productManuals, total } = response.data
|
|
setProductManuals(productManuals)
|
|
setTotalData(total)
|
|
}
|
|
} catch (error) {
|
|
console.error('Failed to fetch product manuals:', error)
|
|
} finally {
|
|
setLoading(false)
|
|
}
|
|
}
|
|
|
|
useEffect(() => {
|
|
getProductManuals()
|
|
}, [currentPage, itemsPerPage])
|
|
|
|
const handleFilterChange = () => {
|
|
setCurrentPage(1)
|
|
getProductManuals()
|
|
}
|
|
|
|
const handlePageChange = (event, page) => {
|
|
setCurrentPage(page)
|
|
}
|
|
|
|
return (
|
|
<Box sx={{ padding: 2 }}>
|
|
{/* Filter Text Field */}
|
|
|
|
{/* Table and Pagination */}
|
|
{loading ? (
|
|
<CircularProgress />
|
|
) : (
|
|
<>
|
|
<TableContainer component={Paper}>
|
|
<Table>
|
|
<TableHead>
|
|
<TableRow>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Name</TableCell>
|
|
<TableCell sx={{ fontWeight: 'bold' }}>Action</TableCell>
|
|
</TableRow>
|
|
</TableHead>
|
|
<TableBody>
|
|
{productManuals.map((manual) => (
|
|
<TableRow key={manual._id}>
|
|
<TableCell>{manual.title}</TableCell>
|
|
<TableCell>
|
|
<Button
|
|
variant="contained"
|
|
color="primary"
|
|
component={Link}
|
|
to={`/product-manual/${manual._id}`}
|
|
>
|
|
View
|
|
</Button>
|
|
</TableCell>
|
|
</TableRow>
|
|
))}
|
|
</TableBody>
|
|
</Table>
|
|
</TableContainer>
|
|
|
|
{/* Pagination */}
|
|
<Box sx={{ display: 'flex', justifyContent: 'center', mt: 2 }}>
|
|
<Pagination
|
|
count={Math.ceil(totalData / itemsPerPage)}
|
|
page={currentPage}
|
|
onChange={handlePageChange}
|
|
color="primary"
|
|
/>
|
|
</Box>
|
|
</>
|
|
)}
|
|
</Box>
|
|
)
|
|
}
|
|
|
|
export default ProductManual
|